In this tutorial, we will learn about how to use GraphQL in magento 2.3.
Magento 2.3 version comes with many new features. For example, MSI (Multi-store Inventory), PWA (Progressive Web Apps), GraphQL and many others.
Before the implementation of GraphQL in Magento2.3 let’s understand its basics.
What is GraphQL?
GraphQL is a query language for APIs which is used to load data from a server to a client. It’s provide a complete and easy details of the data in your API. Using GraphQL, The user can able to make a single call to retrieve required data rather than to construct several REST request to fetch the same data.
You may like also this :
Advantages of GraphQL :
- Declarative Data Fetch
- No Overfetching with GraphQL
- Better Performance
- Flexible and Faster
- Recover Many Resources in a Single Request
Disadvantages of GraphQL :
- Query Complexity
- Cache at Network Level
Queries and Mutations in GraphQL
Queries
A GraphQL query retrieves data from the Magento server in a similar manner as a REST GET call. It helps to reduce over fetching of data. For more details, you can check here.
Mutations
A Mutation queries modify data in the data store and returns a value. It can be used to insert, update, or delete data. For more details, you can check here.
Now, let’s start to create custom module using GraphQL.
1) First of all, Create registration.php at app/code/RH/CustomGraphQl/ and paste the below code :
<?php
/**
* Copyright © Magento, Inc. All rights reserved.
* See COPYING.txt for license details.
*/
/**
* Created By : Rohan Hapani
*/
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'RH_CustomGraphQl',
__DIR__
);
2) After that, Create module.xml at app/code/RH/CustomGraphQl/etc and paste the below code :
<?xml version="1.0"?>
<!--
/**
* Copyright © Magento, Inc. All rights reserved.
* See COPYING.txt for license details.
*
* Created By : Rohan Hapani
*/
-->
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="RH_CustomGraphQl">
<sequence>
<module name="Magento_GraphQl" />
</sequence>
</module>
</config>
This module is depends on Magento_GraphQl module. So, we have set dependency in module.xml file.
Whenever, we create GraphQL module we need to create schema.graphqls file in etc folder of the module. This file defines the structure of queries and mutations and describe that which data can be used for input and output. For more details, you can check from here.
3) After that, Create schema.graphqls at app/code/RH/CustomGraphQl/etc and paste the below code :
# Copyright © Magento, Inc. All rights reserved.
# Created By : Rohan Hapani
type Query {
quoteData (
id: Int @doc(description: "Id of the Quote Data")
): QuoteData @resolver(class: "RH\\CustomGraphQl\\Model\\Resolver\\QuoteData") @doc(description: "The Quote Data Query Returns Data about a Quote Object")
}
type QuoteData @doc(description: "Quote information by quote id") {
base_currency_code: String @doc(description: "Base Currency Code from Quote Object")
grand_total: String @doc(description: "Grand Total of Specific Quote Object items")
customer_name: String @doc(description: "Customer Name from Quote Object")
}
Now, Create a Resolver Model for our custom module. In this model, resolve() method will simply return data of quote as per GraphQL schema type.
4) Then, Create QuoteData.php file at app/code/RH/CustomGraphQl/Model/Resolver folder and paste the below code :
<?php
/**
* Copyright © Magento, Inc. All rights reserved.
* See COPYING.txt for license details.
*
*/
/**
* Created By : Rohan Hapani
*/
declare (strict_types = 1);
namespace RH\CustomGraphQl\Model\Resolver;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Framework\GraphQl\Config\Element\Field;
use Magento\Framework\GraphQl\Exception\GraphQlInputException;
use Magento\Framework\GraphQl\Exception\GraphQlNoSuchEntityException;
use Magento\Framework\GraphQl\Query\ResolverInterface;
use Magento\Framework\GraphQl\Schema\Type\ResolveInfo;
/**
* Quote data field resolver, used for GraphQL request processing
*/
class QuoteData implements ResolverInterface
{
/**
* @var \Magento\Quote\Api\CartRepositoryInterface
*/
protected $quoteRepository;
/**
* @param \Magento\Quote\Api\CartRepositoryInterface $quoteRepository
*/
public function __construct(
\Magento\Quote\Api\CartRepositoryInterface $quoteRepository
) {
$this->quoteRepository = $quoteRepository;
}
/**
* @inheritdoc
*/
public function resolve(
Field $field,
$context,
ResolveInfo $info,
array $value = null,
array $args = null
) {
$quoteId = $this->getQuoteId($args);
$quoteData = $this->getQuoteData($quoteId);
return $quoteData;
}
/**
* @param array $args
* @return int
* @throws GraphQlNoSuchEntityException
*/
private function getQuoteId(array $args): int
{
if (!isset($args['id']))
{
throw new GraphQlInputException(__('"Quote id should be specified'));
}
return (int) $args['id'];
}
/**
* @param int $quoteId
* @return array
* @throws GraphQlNoSuchEntityException
*/
private function getQuoteData(int $quoteId): array
{
try
{
$quote = $this->quoteRepository->get($quoteId);
$quoteData = [
'base_currency_code' => $quote->getBaseCurrencyCode(),
'grand_total' => $quote->getGrandTotal(),
'customer_name' => $quote->getCustomerFirstname() . ' ' . $quote->getCustomerLastname(),
];
}
catch (NoSuchEntityException $e)
{
throw new GraphQlNoSuchEntityException(__($e->getMessage()), $e);
}
return $quoteData;
}
}
Now, just run below commands for install our module :
php bin/magento s:up
php bin/magento s:s:d -f
php bin/magento c:c
In Last, check query output by installing chrome extension ChromeiQL or Postman (GraphQL supported version).
Execute URL : http://127.0.0.1/m231/graphql
Where Base URL : http://127.0.0.1/m231/ and End Point : graphql
In Request Body, You need to pass below data where id is your quote id.
{
quoteData(id: 1) {
base_currency_code
customer_name
grand_total
}
}
Output :
{
"data": {
"quoteData": {
"base_currency_code": "USD",
"grand_total": "36.3900",
"customer_name": "Veronica Costello"
}
}
}
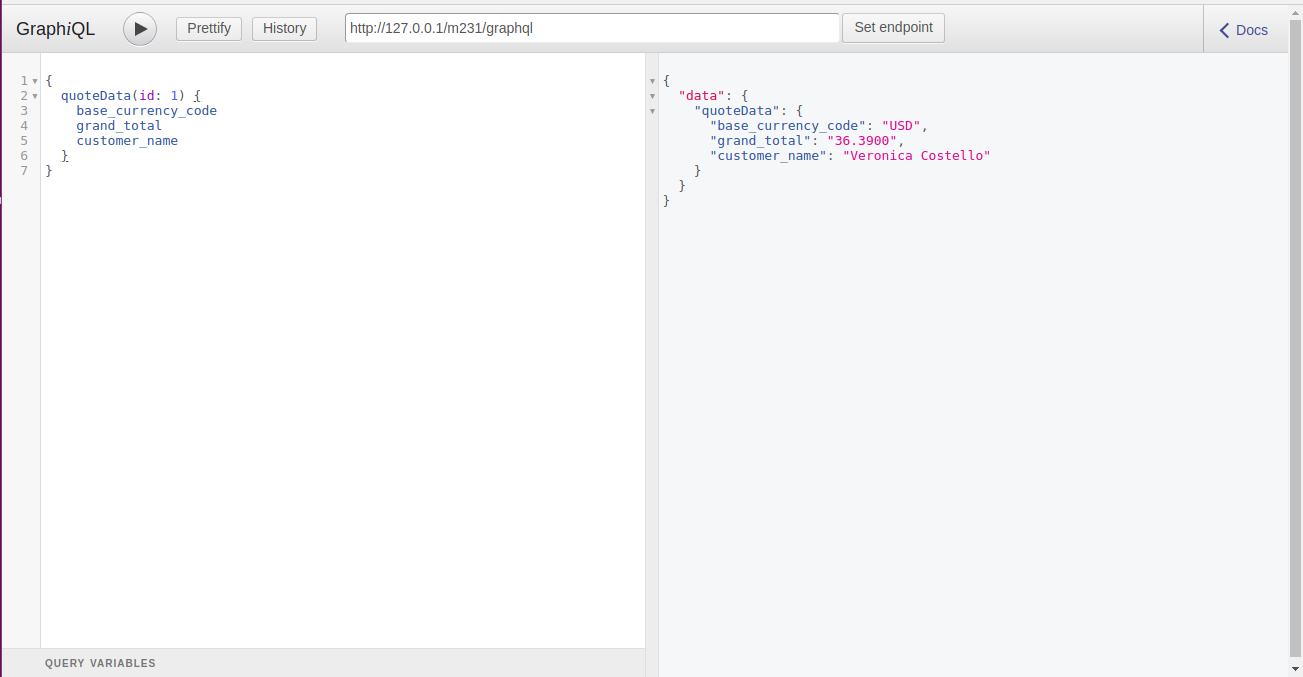