In this article, we will learn about how to get orders list by customer id using GraphQL API in Magento 2.
After Magento 2.3, GraphQL is most popular feature to make it simple to fetch all require data with one single request. With GraphQL, you can send a query to your API and get response what you need. So, Now a days people are prefer to use GraphQL instead of REST API.
Sometimes, user need to fetch sales order list records of customer by customer id from outside Magento. At that time, GraphQL is best alternative to REST and SOAP web APIs.
Let’s now create simple module for get orders information by customer id using GraphQL. Using GraphQL, We can get order information of specific registered customer by customer id.
You may also like this :
1) Firstly, Create registration.php register our module at app/code/RH/CustomerGraphQL/ and paste the below code :
<?php
/**
* Copyright © Magento, Inc. All rights reserved.
* See COPYING.txt for license details.
*/
/**
* Created By : Rohan Hapani
*/
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'RH_CustomerGraphQL',
__DIR__
);
2) Then, Create module.xml file at app/code/RH/CustomerGraphQL/etc/ and paste the below code. Our module depends on GraphQL and Sales module. So, we need to set dependency on module.xml file.
<?xml version="1.0"?>
<!--
/**
* Copyright © Magento, Inc. All rights reserved.
* See COPYING.txt for license details.
*
* Created By : Rohan Hapani
*/
-->
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="RH_CustomerGraphQL" >
<sequence>
<module name="Magento_Sales"/>
<module name="Magento_GraphQl"/>
</sequence>
</module>
</config>
When we create GraphQL module, we must need to contain schema.graphqls file under the etc folder of the module. schema.graphqls is most important file for any GraphQL Query implementation and describe about the data.
3) After, Create schema.graphqls file at app/code/RH/CustomerGraphQL/etc/ and paste the below code :
type Query {
CustomerOrderList (
customer_id: Int @doc(description: "Id of the Customer")
): SalesOrder @resolver(class: "RH\\CustomerGraphQL\\Model\\Resolver\\CustomerOrder") @doc(description: "The Sales Order query returns information about a customer placed order")
}
type SalesOrder @doc(description: "Sales Order graphql gather data of order item information") {
fetchRecords : [CustomerOrderRecord] @doc(description: "An array of customer placed order fetch records")
}
type CustomerOrderRecord @doc(description: "Customer placed order items information") {
increment_id: String @doc(description: "Increment Id of Sales Order")
customer_name: String @doc(description: "Customer name of Sales Order")
grand_total: String @doc(description: "Grand total of Sales Order")
qty: Int @doc(description: "Order item quantity")
shipping_method: String @doc(description: "Shipping method of order placed")
}
In that file, we will pass customer_id for get customer id as input. We have created CustomerOrderRecord array for sales order information which we required in the response of query. You can customize field based on your requirements.
4) At last, Create CustomerOrder.php Resolver file at app/code/RH/CustomerGraphQL/Model/Resolver/ and paste the below code :
<?php
/**
* Created By : Rohan Hapani
*/
declare (strict_types = 1);
namespace RH\CustomerGraphQL\Model\Resolver;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Framework\GraphQl\Config\Element\Field;
use Magento\Framework\GraphQl\Exception\GraphQlInputException;
use Magento\Framework\GraphQl\Exception\GraphQlNoSuchEntityException;
use Magento\Framework\GraphQl\Query\ResolverInterface;
use Magento\Framework\GraphQl\Schema\Type\ResolveInfo;
/**
* Customer order sales field resolver, used for GraphQL request processing
*/
class CustomerOrder implements ResolverInterface {
/**
* @var \Magento\Sales\Api\OrderRepositoryInterface
*/
protected $orderRepository;
/**
* @var \Magento\Framework\Api\SearchCriteriaBuilder
*/
protected $searchCriteriaBuilder;
/**
* @var \Magento\Framework\Api\SearchCriteriaBuilder
*/
protected $priceCurrency;
/**
* @param \Magento\Sales\Api\OrderRepositoryInterface $orderRepository
* @param \Magento\Framework\Api\SearchCriteriaBuilder $searchCriteriaBuilder
* @param \Magento\Framework\Pricing\PriceCurrencyInterface $priceCurrency
*/
public function __construct(
\Magento\Sales\Api\OrderRepositoryInterface $orderRepository,
\Magento\Framework\Api\SearchCriteriaBuilder $searchCriteriaBuilder,
\Magento\Framework\Pricing\PriceCurrencyInterface $priceCurrency
) {
$this->orderRepository = $orderRepository;
$this->searchCriteriaBuilder = $searchCriteriaBuilder;
$this->priceCurrency = $priceCurrency;
}
/**
* @inheritdoc
*/
public function resolve(
Field $field,
$context,
ResolveInfo $info,
array $value = null,
array $args = null
) {
$customerId = $this->getCustomerId($args);
$customerOrderData = $this->getCustomerOrderData($customerId);
return $customerOrderData;
}
/**
* @param array $args
* @return int
* @throws GraphQlInputException
*/
private function getCustomerId(array $args): int {
if (!isset($args['customer_id'])) {
throw new GraphQlInputException(__('Customer id should be specified'));
}
return (int) $args['customer_id'];
}
/**
* @param int $customerId
* @return array
* @throws GraphQlNoSuchEntityException
*/
private function getCustomerOrderData(int $customerId): array {
try {
$searchCriteria = $this->searchCriteriaBuilder->addFilter('customer_id', $customerId, 'eq')->create();
$orderList = $this->orderRepository->getList($searchCriteria);
$customerOrder = [];
foreach ($orderList as $order) {
$order_id = $order->getId();
$customerOrder['fetchRecords'][$order_id]['increment_id'] = $order->getIncrementId();
$customerOrder['fetchRecords'][$order_id]['customer_name'] = $order->getCustomerFirstname() . ' ' . $order->getCustomerLastname();
$customerOrder['fetchRecords'][$order_id]['grand_total'] = $this->priceCurrency->convertAndFormat($order->getGrandTotal(), false);
$customerOrder['fetchRecords'][$order_id]['qty'] = $order->getTotalQtyOrdered();
$customerOrder['fetchRecords'][$order_id]['shipping_method'] = $order->getShippingMethod();
}
} catch (NoSuchEntityException $e) {
throw new GraphQlNoSuchEntityException(__($e->getMessage()), $e);
}
return $customerOrder;
}
}
Now, execute this below commands
php bin/magento s:up
php bin/magento s:s:d -f
php bin/magento c:c
Now, You can check output in ChromeiQL addons or Postman (GraphQL supported version).
In Request Body, you need to pass query required data.
{
CustomerOrderList (customer_id: 1) {
fetchRecords{
increment_id
customer_name
grand_total
qty
shipping_method
}
}
}
We have passed customer id as 1 for get order information.
Result :
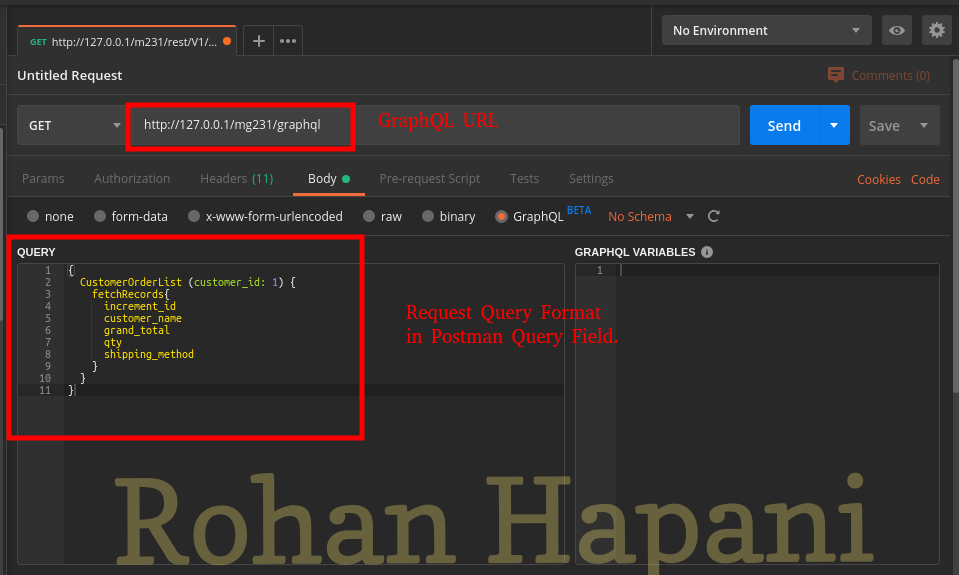
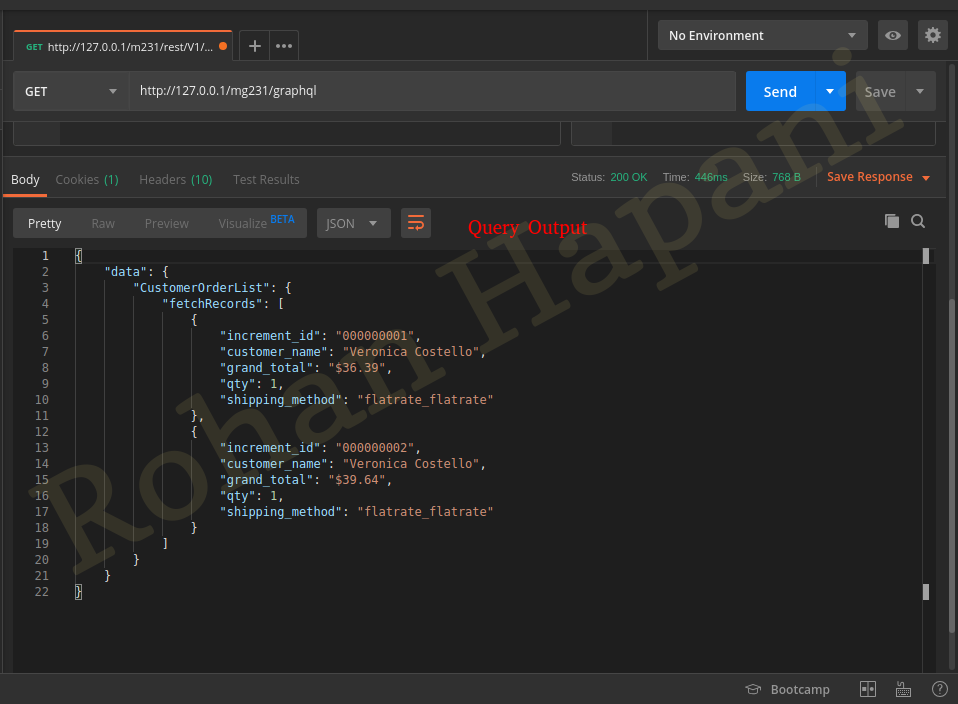
I hope this blog will helpful for easily understand about get orders list by customer id using GraphQL in Magento 2. In case, I missed anything or need to add some information, always feel free to leave a comment in this blog, I’ll get back with proper solution